Reactreact-native
React ContextAPI
By Fangtai Dong

- Published on
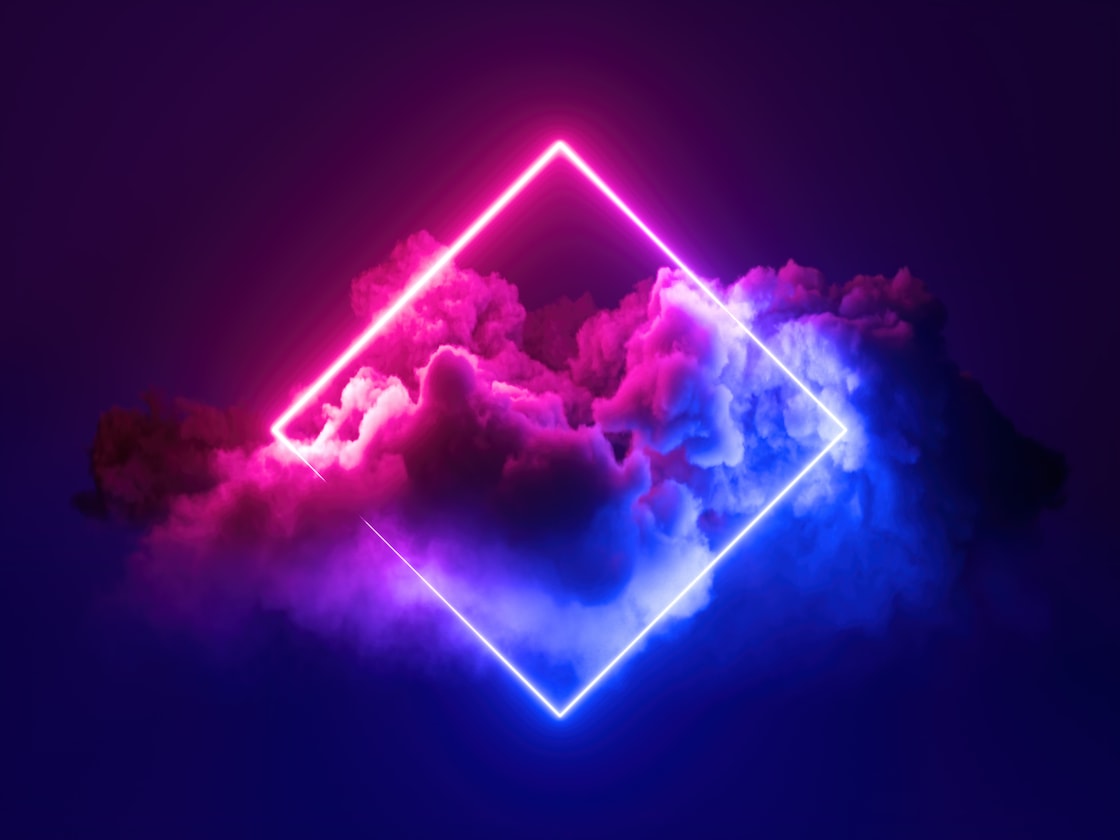
Sharing
Theme
Related to React contextAPI
React's Context API allows data to be passed more easily in the component tree without having to be passed step by step through each individual component(props drilling). The following is a simple and easy-to-understand example, through which you can see how to use Context to pass data.
1. Create Context
import React, { createContext, useState } from 'react'
const ThemeContext = createContext()
2. Create Provider Component
export const ThemeProvider = ({ children }) => {
const [theme, setTheme] = useState('dark')
const toggleTheme = () => {
setTheme((prevTheme) => (prevTheme === 'dark' ? 'light' : 'dark'))
}
return <ThemeContext.Provider value={{ theme, toggleTheme }}>{children}</ThemeContext.Provider>
}
3. Export Context
export default ThemeContext
4. Use ThemeContext in your component
import React, { useContext } from 'react'
import ThemeContext from './ThemeContext'
const ThemedComponent = () => {
const { theme, toggleTheme } = useContext(ThemeContext)
return (
<div>
<p>Current Theme is: {theme}</p>
<button onClick={toggleTheme}>Toggle Theme!</button>
</div>
)
}
export default ThemedComponent
5. Put ThemeProvider into App.js
import React from 'react';
import { ThemeProvider } from './ThemeContext';
import ThemedComponent from './ThemedComponent';
function App() {
return (
<ThemeProvider>
<div className="App">
<ThemedComponent />
</div>
</ThemeProvider>
);
}
export default App;
Conclusion
In this example, the ThemeProvider
component is at the top of the application, which provides theme
and toggleTheme
functions for all the lower components. Then, we use useContext
in the ThemedComponent
component to get the current theme and switching functions. In this way, these values can be obtained regardless of the level of ThemedComponent
, without passing props step by step.
Hi there! Want to support my work?