React & React Native
By Fangtai Dong

- Published on
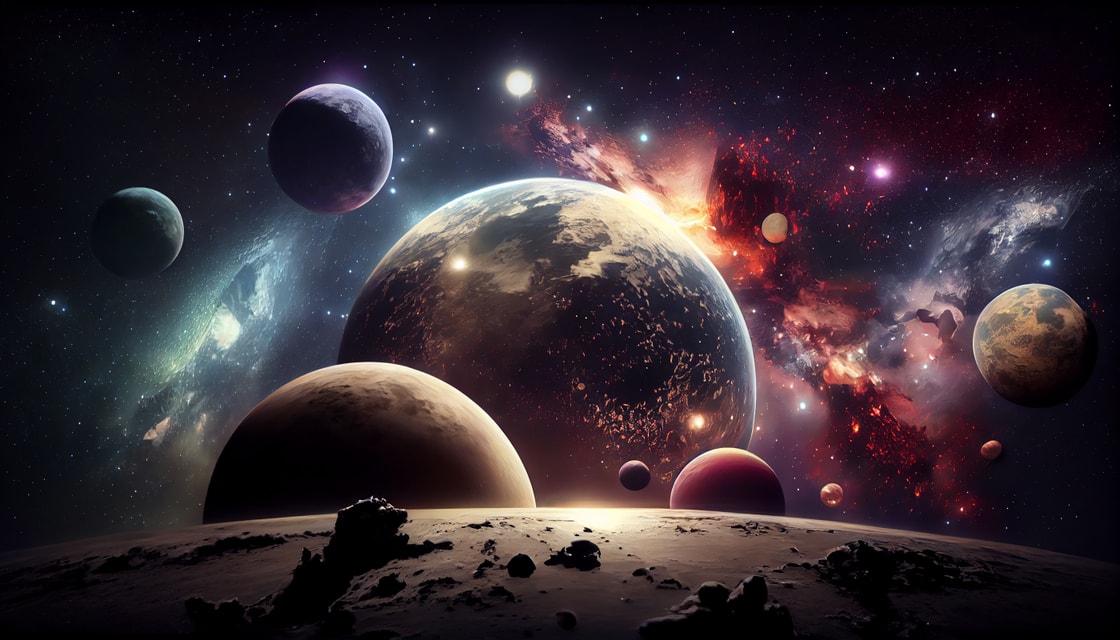
Sharing
React Native
Basics
- Core Components
- Styling
- FlexBox -- Elements are positioned inside of containers
- Interactivity
import { useState } from 'react' // useState hook to get users' every keystroke export default function App() { const [Text, setText] = useState('') const inputHandler = () => {} return <View style={styles.appContainer} /> } const styles = StyleSheet.create({ appContainer: {}, })
- ScrollView Component
- FlatList Component
<FlatList data={courseGoals} renderItem={(itemData) => { return ( <View style={styles.goalItem}> <Text style={styles.goalText}> {itemData.item.text} </Text> </View> ) }} keyExtractor={(item, index) => { return item.id }} alwaysBounceVertical={false} />
- Splitting Components
import { StyleSheet, View, Text } from 'react-native'
- Props Passing
- State Bind Input Value Property
- Pressable Component (Children)
<Pressable onPress={props.onDeleteItem.bind(this, props.id)}></Pressable>
- In the App Component (Parent)
const handleDelete(id) { setGoals((currentGoals) => { return currentGoals.filter(goal => goal.id !== id ); }) } //... <GoalItem text={itemData.item.text} id={itemData.item.id} onDeleteItem={handleDelete} ></GoalItem>
- Android Ripple Effect & IOS Alternative
<Pressable android_ripple={{color: 'red'}} style={({pressed}) => pressed && styles.pressedItem} onPress={props.onDeleteItem.bind(this, props.id) ></Pressable>
- Adding a Modal Screen
import { Modal } from 'react-native'
- 2 Props in Modal Component
<Modal visible={props.visible} animationType="slide"></Modal>
- Image Component
import { Image } from 'react-native' <Image style={styles.image} // relative path source={require('../assets/images/goals.png')}/>
- Status Bar
import { StatusBar } from 'expo-status-bar' <StatusBar style="light" />
Advance
- React Native use Hermes engine(instead of JavaScript Core) to improve its performance on Andriod.
- Expo -- It is a framework which makes React Native Development simple
- Sign up an Expo account
- Create a Expo snack
- Coding logic
- Folder Structure
- App.js
- src
- utils
- features -- entry point for the features that I want to build
- components
- SafeAreaView Component
- Platform and StatusBar Component
StyleSheet.create({ container: { flex:1, paddingTop: Platform.OS === 'android'? StatusBar.currentHeight : 0; } })
- React Native Paper
- Check the latest version and update it in package.json
- Storing A Subject
- Think about: How can I store user input in R.N? 🤔️
- Use shorthand
import { TextInput } from 'react-native-paper'; //... const [subject, setSubject] = useState(null); //... onChangeText={setSubject} is a shorthand of //... onChangeText={(val)=>setSubject(val)} <TextInput onChangeText={setSubject} label="Please Input" />
- Custom Components import from components folder
- Child Event to Parent Component
- Parent Component:
App
const [currentSubject,setCurrentSubject] = useState(null); //... return ( {!currentSubject ? <Focus addSubject={setCurrentSubject}/> : <View><Text>Other Page</Text></View> } )
- Child Component:
<Focus />
const Focus = ({addSubject}) => { //... return ( <RoundedButton onPress={()=>{addSubject(subject)}}/> ) }
- useState VS useRef
- useState will cause a re-render
- useRef will not
- only return one object in which I can set it to what variable I want.
- Track the value of setInterval
- useEffect
- only execute once for the 1st moment
useEffect(()=>{console.log('Effect Running')}, []);
- 1st moment and every enteredPassword state update will run it
useEffect( ()=>{console.log('Effect Running')}, [enteredPassword]);
- Clean up function will run every 2nd time component re-executed based on the enteredPassword state change. And this clean up function will run before the effect function run.
- Clean up function will also run when the component is removed.
useEffect( ()=>{console.log('Effect Running') return ()=>{console.log('Clean Up')} }, [enteredPassword])
- CountDown Function Component
- Think about how do create this component? 🤔️
- ProgressBar
- Import from react native paper
- progress prop in this component
- Hook up the value by using useState
const [progress, setProgress] = useState(1);
//...
<Progress progress={progress}/>
- Think about the fallback function in CountDown component 🤔️
<CountDown onProgress={(prog)=>setProgress(prog)}/>
- shorthand writting (get value & set value) in callback function
<CountDown onProgress={setProgress}/>
- Vibration
- import from 'react-native'
- Pass a function as a parameter to component's prop
- useKeepAwake hook imported from 'expo-keep-awake'
- FlatList
- data =
{}
- renterItem =
{}
- keyExtractor =
{}
Local Setup
- Expo export
- Git
- Node.js
- Yarn or npm
- Yarn is more reliable with React Native project since they all developed by FB
- cd project folder and run
- yarn
- sudo npm install -g expo-cli
- cat package.json
- yarn ios
- Xcode
- (Applications --> Xcode --> Contents --> Developer --> Applications --> Simulator)
- Android Studio
- VS code
- Key chain management
- Imposter syndrome --> sometime is good for learning 🤔️
Hi there! Want to support my work?